HTTP Services
As Claro provides simple HTTP support out of the box, you can export HTTP Service definitions from Module APIs. For example, the following Buggy Buggies game1 can be controlled by sending HTTP GET requests to the endpoints defined below:
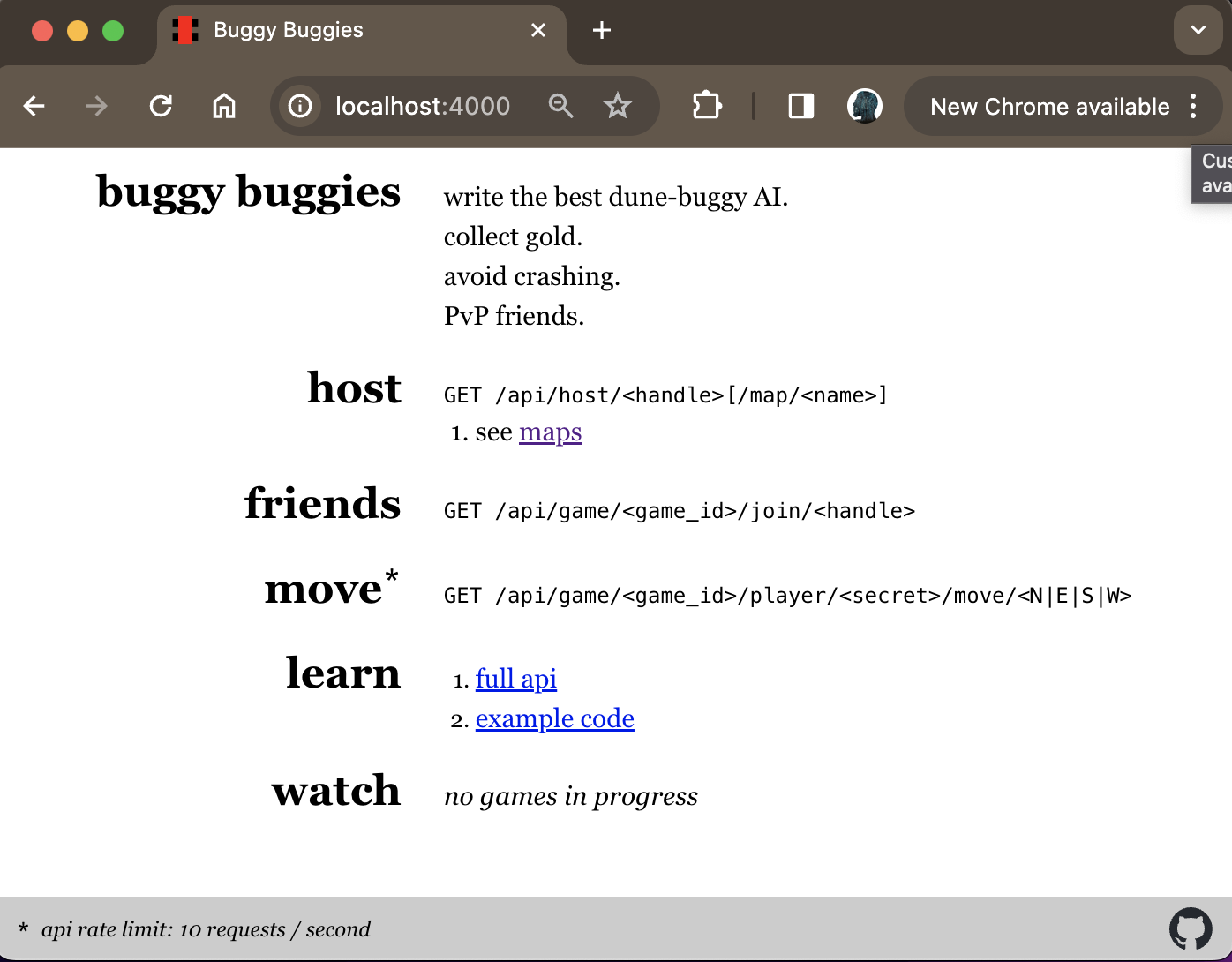
So, we can encode this api as an HTTP Service that can be called programmatically by Claro programs by exporting the following from a Module API:
Fig 1:
# ex1.claro_module_api
# This encodes the public API of the Buggy-Buggies HTTP service.
# Claro will generate a non-blocking RPC client for you via the following:
# `var myClient: HttpClient<BuggyBuggies> = http::getHttpClient("https://buggy-buggies.gigalixirapp.com");`
HttpService BuggyBuggies {
hostGame: "/api/host/{handle}",
friendsJoin: "/api/game/{gameId}/join/{handle}",
move: "/api/game/{gameId}/player/{secret}/move/{direction}",
worldInfo: "/api/game/{gameId}/player/{secret}/info",
reset: "/api/game/{gameId}/player/{secret}/reset"
}
# Now there's a single static definition of which client will be used for sending reqs to the Buggy Buggies server.
static BUGGY_BUGGIES_CLIENT: HttpClient<BuggyBuggies>;
In this case the static HTTP client being exported by this Module will allow messages to be sent to the local port that the Buggy Buggies game is running on:
Fig 2:
# ex1-impl.claro
provider static_BUGGY_BUGGIES_CLIENT() -> HttpClient<BuggyBuggies> {
# This client will send reqs to localhost but could use a public endpoint.
return http::getHttpClient("http://localhost:4000");
}
Now, a dependent can easily make calls to the various HTTP endpoints exposed by the service and from the dependent's
perspective it appears just like any other procedure call returning a future<...>
.
Fig 3:
var hostGameRes: oneof<string, std::Error<string>>
<-| BuggyBuggies::hostGame(BuggyBuggies::BUGGY_BUGGIES_CLIENT, "jason");
print("Host Game Response:\n{hostGameRes}");
Output:
Host Game JSON Response:
{"reason":null,"success":true,"result":{"watch":"http://localhost:4000/game/04cfad01","secret":"7f3b8ae5","game_id":"04cfad01","example":"curl -X GET http://localhost:4000/api/game/04cfad01/player/7f3b8ae5/move/N"}}
Now, you can imagine how a more complex Claro program could use the HTTP client to this service to make a bot that automatically plays the game collecting as much gold as possible.
Check out the full-fledged implementation of the Claro program controlling the buggy in the above gif.
Buggy Buggies was built by Jake Wood, thank you for sharing this!